1. What is byte-code? What is its importance?
2. What is the syntax and semantics of a programming language?
3. What steps must the programmer take to create an executable Java program?
4. List the primitive data types Java supports. Indicate the kind of values each type can store.
1. How is Define high-level languages, machine language and low-level language. Explain how the languages correspond to one another.
2. the % (modulus) operator used? What is the common use of the modulus operator?
3. Explain the difference between an implicit type cast and an explicit type cast.
4. Write a Java statement to access the 7th character in the String variable myString and place it in the char variable c.
5. Write a Java statement to determine the length of a string variable called input. Store the result in an integer variable called strLength.
6. Why is using named constants a good programming practice?
7. How are line comments and block comments used?
What are the values of the variables a, b, c, and d after the execution of the following expressions?
int a = 3;
int b = 12;
int c = 6;
int d = 1;
d = d * a;
c = c + 2 * a;
d = d – b / c;
c = c * b % c;
b = b / 2;
What is the output produced by the following lines of code?
int value1 = 3;
int value2 = 4;
int result = 0;
result = value1++ * value2–;
System.out.println(“Post increment/decrement: ” + result);
Practical Exercise 1
Write a program that starts with the string variable first set to your first name and the string variable last set to your last name. Both names should be all lowercase. Your program should then create a new string that contains your full name in pig latin with the first letter capitalized for the first and last name. Use only the rule of moving the first letter to the end of the word and adding “ay”. Finally output the pig latin name to the screen. Use the subString and toUpperCase methods to construct the new name.
first = “walt”;
last = “savitch”;
would output “Altway Avitchsay”
Practical Exercise 2.
A simple rule to estimate your ideal body weight is to allow 110 pounds for the first 5 feet of height and 5 pounds for each additional inch. Write a program with a variable for the height of a person in feet and another variable for the additional inches. Assume the person is at least 5 feet tall. For example a person that is 6 feet and 3 inches tall would be represented with a variable that stores the number 6 and another variable that stores the number 3. Based on these values, calculate and output the ideal body weight.
1. Write a Java statement to display your name in the console window.
2. Write ONE Java statement to display your first and last name on two separate lines.
3. Write Java statements to apply currency formatting to the number 100. Indicate the package you need to import.
4. Write a Java program to create and display 57.32% using the DecimalFormat class. Include the necessary import statement to use the DecimalFormat class.
5. Explain the significance of the pattern string used by the DecimalFormat object, and give an example of a valid pattern.
6. What does it mean to prompt the user?
7. Why is echoing user input a good programming practice?
8. If there is no loss of efficiency in importing an entire Java package instead of importing only classes you use into your program, why would you not just import the entire package?
9. Discuss the differences between the break and the continue statements when used in looping mechanisms.
10. Write a complete Java program that prompts the user for a series of numbers to determine the smallest value entered. Before the program terminates, display the smallest value.
11. Write a complete Java program that uses a for loop to compute the sum of the even numbers and the sum of the odd numbers between 1 and 25.
12. What is short-circuit evaluation of Boolean expressions?
13. Discuss the rules Java follows when evaluating expressions.
14. Write Java code that uses a do while loop that prints even numbers from 2 through 10.
15. Write Java code that uses a while loop to print even numbers from 2 through 10.
16. What is a sentinel value and how is it used?
17. Write Java code that uses a for statement to sum the numbers from 1 through 50. Display the total sum to the console.
18. What is the output of the following code segment?
public static void main(String[] args) {
int x = 5;
System.out.println(“The value of x is:” + x);
while(x > 0) {
x++;
}
System.out.println(“The value of x is:” + x);
}
Practical Exercises on Console IO
(To Be Done In Practical With Partner)
Practical Exercise 1.
The straight line method for computing the yearly depreciation in value D for an item is given by the following formula:
D = (P – S)/Y
Where P is the purchase price, S is the salvage value, and Y is the number of years the item is used. Write a program that takes as input the purchase price of an item, the expected number of years of service, and the expected salvage value. The program should then output the yearly depreciation for the item.
Practical Exercise 2.
Create a text file (using Eclipse) that contains the text “I hate typing up stuff from the textbook”. Write a program that reads in this line of text from the file. The word hate is changed to love and output to the screen. This should handle any sentence containing hate and should handle the first incidence of hate.
Some Hints:
You will need to use some skills you have not got yet.
Firstly reading from a file.
If you recall you usually use
Scanner file = new Scanner(System.in);
To read from a keyboard. The System.in is a stream that connects to the keyboard
In this question you want to connect to a file on the disk.
Consequently you replace System.in with new File(“file.txt”) This will open a stream
to the file file.txt on your disk.
Second error handling
Because reading from a disk can have unexpected problems we need to handle errors that might occur
Wrap everything in your main method with an exception handling clause.
class FileIO {
public static void main(String[] argv) {
try {
//YOUR CODE GOES HERE.
} catch (Exception ex) {System.out.println(“File Not Found”);}
}
}
We will cover how this works later in the semester
Practical Exercise 3
The game of Pig is a simple two player dice game in which the first player to reach 100 or more points wins. Player takes turns. On each turn, a player rolls a six sided die:
* If the player rolls a 1, then the player gets no new points and it becomes the other players turn.
* If the player rolls 2 through 6 then he or she either
– roll again or,
– hold. At this point, the sum of all rolls is added to the players score and it becomes the other players turn.
Write a program that plays the game of Pig, where one player is a human and the other is the computer. When it is the humans turn, the program should show the score of both players and the previous roll. Allow the human to input r to roll again or h to hold.
The computer should play according to the following rule:
* Keep rolling when it is the computers turn until it has accumulated 20 or more points, then hold. If the computer wins or rolls a 1, then the turn ends immediately.
1. Write a Java method that prints the phrase “Five purple people eaters were seen munchingMartians”.
2. What is the purpose of the new operator?
3. Write a Java method that returns the value of PI, where PI = 3.1415926535.
4. Define the terms arguments and parameters. How are they different?
5. Write a method called power the computes xn where x and n and positive integers. The method has two integer parameters and returns a value of type long.
6. Write a method called Greeting that displays a personalized greeting given a first name.
7. Discuss a situation in which it is appropriate to use the this parameter.
8. Write a method called isEqual that returns a Boolean value. The method compares two integers for equality.
9. Discuss the public and private modifiers in context of methods and instance variables.
10. Create a class named Appointment that contains instance variables startTime, endTime, dayOfWeek (valid values are Sunday through Saturday), and a date which consists of a month, day and year. All times should be in military time, therefore it is appropriate to use integers to represent the time. Create the appropriate accessor and mutator methods.
11. Discuss the importance of accessor and mutator methods and how they apply to the abstraction concept.
12. Write a complete Java class that uses the console window to prompt the user for a sentence to parse. Use the StringTokenizer class to echo the tokens back to the console.
13. Write a Java class that represents a Student with instance variables name, id, and gpa. Include constructors, accessor, mutator and any facilitator methods you may need.
14. Write a driver program to test your Student class created in the previous question.
Practical Exercise 1
Write a program that outputs the lyrics for “Ninety-nine Bottles of beer on the Wall.” Your program should print the number of the bottles in english not as a number. For example
Ninety-nine bottles of beer on the wall.
…
One bottle of beer on the wall.
One bottle of beer,
Take one down, pass it around,
Zero bottles of beer on the wall.
Your program should not just have ninety nine output statements.
Practical Exercise 2
Define a class whose objects are records o n animal species. The class should have instance variables for the species name, population, and growth rate. The growth rate is a percentage that can be positive or negative and can exceed 100%. Include a suitable collection of constructors , mutators methods ands accessor methods. Include a toString method and an equals method. Include a boolean value method named endangered that returns true when the growth rate is negative and returns false otherwise. Write a test program (or programs) that tests each method in your class definition.
Practical Exercise 3
Create a class named Pizza that stores information about a single pizza. It should contain the following.
* (either small medium or large) , the number of cheese toppings, the number of pepperoni toppings, and the number of ham toppings.
* constructor(s) that set all the instance variables.
* public methods to get and set the instance variables.
* public method named calcCost() that returns a double that is the cost of the pizza.
Pizza cost is determined by:
Small $10 + $2 per topping
Medium $12 + $2 per topping
Large $14 + $2 per topping
* A public method named getDescription() that returns a String containing the pizza size, quantity or each topping, the pizza cost as calculated by calcCost().
Write test code to create several pizzas and output their descriptions. For example, a large pizza with one cheese, one pepperoni and two ham toppings should cost a total of $22.
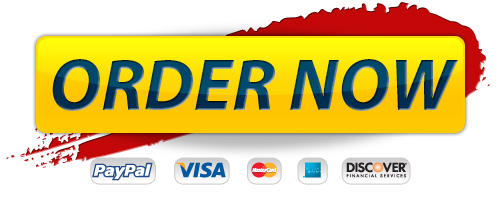
Order Management

Premium Service
- 100% Custom papers
- Any delivery date
- 100% Confidentiality
- 24/7 Customer support
- The finest writers & editors
- No hidden charges
- No resale promise
Format and Features
- Approx. 275 words / page
- All paper formats (APA, MLA, Harvard, Chicago/Turabian)
- Font: 12 point Arial/Times New Roman
- Double and single spacing
- FREE bibliography page
- FREE title page
0% Plagiarism
We take all due measures in order to avoid plagiarisms in papers. We have strict fines policy towards those writers who use plagiarisms and members of QAD make sure that papers are original.